Initial HeatpumpIR library, refactored from my 'arduino-wp-heatpump-controller' repository
This commit is contained in:
commit
d5d00a8686
|
@ -0,0 +1,123 @@
|
|||
#include <Arduino.h>
|
||||
#include "CarrierHeatpumpIR.h"
|
||||
|
||||
CarrierHeatpumpIR::CarrierHeatpumpIR()
|
||||
{
|
||||
}
|
||||
|
||||
void CarrierHeatpumpIR::send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
// Sensible defaults for the heat pump mode
|
||||
|
||||
byte operatingMode = CARRIER_AIRCON1_MODE_HEAT;
|
||||
byte fanSpeed = CARRIER_AIRCON1_FAN_AUTO;
|
||||
byte temperature = 23;
|
||||
|
||||
if (powerModeCmd == POWER_OFF)
|
||||
{
|
||||
operatingMode = CARRIER_AIRCON1_MODE_OFF;
|
||||
}
|
||||
else
|
||||
{
|
||||
switch (operatingModeCmd)
|
||||
{
|
||||
case MODE_AUTO:
|
||||
operatingMode = CARRIER_AIRCON1_MODE_AUTO;
|
||||
break;
|
||||
case MODE_HEAT:
|
||||
operatingMode = CARRIER_AIRCON1_MODE_HEAT;
|
||||
break;
|
||||
case MODE_COOL:
|
||||
operatingMode = CARRIER_AIRCON1_MODE_COOL;
|
||||
break;
|
||||
case MODE_DRY:
|
||||
operatingMode = CARRIER_AIRCON1_MODE_DRY;
|
||||
break;
|
||||
case MODE_FAN:
|
||||
operatingMode = CARRIER_AIRCON1_MODE_FAN;
|
||||
temperatureCmd = 22; // Temperature is always 22 in FAN mode
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
switch (fanSpeedCmd)
|
||||
{
|
||||
case FAN_AUTO:
|
||||
fanSpeed = CARRIER_AIRCON1_FAN_AUTO;
|
||||
break;
|
||||
case FAN_1:
|
||||
fanSpeed = CARRIER_AIRCON1_FAN1;
|
||||
break;
|
||||
case FAN_2:
|
||||
fanSpeed = CARRIER_AIRCON1_FAN2;
|
||||
break;
|
||||
case FAN_3:
|
||||
fanSpeed = CARRIER_AIRCON1_FAN3;
|
||||
break;
|
||||
case FAN_4:
|
||||
fanSpeed = CARRIER_AIRCON1_FAN4;
|
||||
break;
|
||||
case FAN_5:
|
||||
fanSpeed = CARRIER_AIRCON1_FAN5;
|
||||
break;
|
||||
}
|
||||
|
||||
if ( temperatureCmd > 16 && temperatureCmd < 31)
|
||||
{
|
||||
temperature = temperatureCmd;
|
||||
}
|
||||
|
||||
sendCarrier(IR, operatingMode, fanSpeed, temperature);
|
||||
}
|
||||
|
||||
// Send the Carrier code
|
||||
// Carrier has the LSB and MSB in different format than Panasonic
|
||||
|
||||
void CarrierHeatpumpIR::sendCarrier(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature)
|
||||
{
|
||||
byte sendBuffer[9] = { 0x4f, 0xb0, 0xc0, 0x3f, 0x80, 0x00, 0x00, 0x00, 0x00 }; // The data is on the last four bytes
|
||||
|
||||
static const prog_uint8_t temperatures[] PROGMEM = { 0x00, 0x08, 0x04, 0x0c, 0x02, 0x0a, 0x06, 0x0e, 0x01, 0x09, 0x05, 0x0d, 0x03, 0x0b };
|
||||
byte checksum = 0;
|
||||
|
||||
// PROGMEM arrays cannot be addressed directly, see http://forum.arduino.cc/index.php?topic=106603.0
|
||||
sendBuffer[5] = pgm_read_byte(&(temperatures[(temperature-17)]));
|
||||
|
||||
sendBuffer[6] = operatingMode | fanSpeed;
|
||||
|
||||
// Checksum
|
||||
|
||||
for (int i=0; i<8; i++) {
|
||||
checksum += IR.bitReverse(sendBuffer[i]);
|
||||
}
|
||||
|
||||
sendBuffer[8] = IR.bitReverse(checksum);
|
||||
|
||||
// 40 kHz PWM frequency
|
||||
IR.setFrequency(40);
|
||||
|
||||
// Header
|
||||
IR.mark(CARRIER_AIRCON1_HDR_MARK);
|
||||
IR.space(CARRIER_AIRCON1_HDR_SPACE);
|
||||
|
||||
// Payload
|
||||
for (int i=0; i<sizeof(sendBuffer); i++) {
|
||||
IR.sendIRByte(sendBuffer[i], CARRIER_AIRCON1_BIT_MARK, CARRIER_AIRCON1_ZERO_SPACE, CARRIER_AIRCON1_ONE_SPACE);
|
||||
}
|
||||
|
||||
// Pause + new header
|
||||
IR.mark(CARRIER_AIRCON1_BIT_MARK);
|
||||
IR.space(CARRIER_AIRCON1_MSG_SPACE);
|
||||
|
||||
IR.mark(CARRIER_AIRCON1_HDR_MARK);
|
||||
IR.space(CARRIER_AIRCON1_HDR_SPACE);
|
||||
|
||||
// Payload again
|
||||
for (int i=0; i<sizeof(sendBuffer); i++) {
|
||||
IR.sendIRByte(sendBuffer[i], CARRIER_AIRCON1_BIT_MARK, CARRIER_AIRCON1_ZERO_SPACE, CARRIER_AIRCON1_ONE_SPACE);
|
||||
}
|
||||
|
||||
// End mark
|
||||
IR.mark(CARRIER_AIRCON1_BIT_MARK);
|
||||
IR.space(0);
|
||||
}
|
|
@ -0,0 +1,44 @@
|
|||
/*
|
||||
Carrier 42NQV035G / 38NYV035H2 heatpump control (remote control P/N WH-L05SE)
|
||||
*/
|
||||
#ifndef CarrierHeatpumpIR_h
|
||||
#define CarrierHeatpumpIR_h
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
#include "HeatpumpIR.h"
|
||||
|
||||
// Carrier (42NQV035G / 38NYV035H2) timing constants (remote control P/N WH-L05SE)
|
||||
#define CARRIER_AIRCON1_HDR_MARK 4320
|
||||
#define CARRIER_AIRCON1_HDR_SPACE 4350
|
||||
#define CARRIER_AIRCON1_BIT_MARK 500
|
||||
#define CARRIER_AIRCON1_ONE_SPACE 1650
|
||||
#define CARRIER_AIRCON1_ZERO_SPACE 550
|
||||
#define CARRIER_AIRCON1_MSG_SPACE 7400
|
||||
|
||||
// Carrier codes
|
||||
#define CARRIER_AIRCON1_MODE_AUTO 0x00 // Operating mode
|
||||
#define CARRIER_AIRCON1_MODE_HEAT 0xC0
|
||||
#define CARRIER_AIRCON1_MODE_COOL 0x80
|
||||
#define CARRIER_AIRCON1_MODE_DRY 0x40
|
||||
#define CARRIER_AIRCON1_MODE_FAN 0x20
|
||||
#define CARRIER_AIRCON1_MODE_OFF 0xE0 // Power OFF
|
||||
#define CARRIER_AIRCON1_FAN_AUTO 0x00 // Fan speed
|
||||
#define CARRIER_AIRCON1_FAN1 0x02
|
||||
#define CARRIER_AIRCON1_FAN2 0x06
|
||||
#define CARRIER_AIRCON1_FAN3 0x01
|
||||
#define CARRIER_AIRCON1_FAN4 0x05
|
||||
#define CARRIER_AIRCON1_FAN5 0x03
|
||||
|
||||
|
||||
class CarrierHeatpumpIR : public HeatpumpIR
|
||||
{
|
||||
public:
|
||||
CarrierHeatpumpIR();
|
||||
void send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd);
|
||||
|
||||
private:
|
||||
void sendCarrier(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature);
|
||||
};
|
||||
|
||||
#endif
|
|
@ -0,0 +1,117 @@
|
|||
#include <Arduino.h>
|
||||
#include "FujitsuHeatpumpIR.h"
|
||||
|
||||
FujitsuHeatpumpIR::FujitsuHeatpumpIR()
|
||||
{
|
||||
}
|
||||
|
||||
void FujitsuHeatpumpIR::send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
// Sensible defaults for the heat pump mode
|
||||
|
||||
byte operatingMode = FUJITSU_AIRCON1_MODE_HEAT;
|
||||
byte fanSpeed = FUJITSU_AIRCON1_FAN_AUTO;
|
||||
byte temperature = 23;
|
||||
|
||||
if (powerModeCmd == POWER_OFF)
|
||||
{
|
||||
operatingMode = FUJITSU_AIRCON1_MODE_OFF;
|
||||
}
|
||||
else
|
||||
{
|
||||
switch (operatingModeCmd)
|
||||
{
|
||||
case MODE_AUTO:
|
||||
operatingMode = FUJITSU_AIRCON1_MODE_AUTO;
|
||||
break;
|
||||
case MODE_HEAT:
|
||||
operatingMode = FUJITSU_AIRCON1_MODE_HEAT;
|
||||
break;
|
||||
case MODE_COOL:
|
||||
operatingMode = FUJITSU_AIRCON1_MODE_COOL;
|
||||
break;
|
||||
case MODE_DRY:
|
||||
operatingMode = FUJITSU_AIRCON1_MODE_DRY;
|
||||
break;
|
||||
case MODE_FAN:
|
||||
operatingMode = FUJITSU_AIRCON1_MODE_FAN;
|
||||
// When Fujitsu goes to FAN mode, it sets the low bit of the byte with the temperature. What is the meaning of that?
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
switch (fanSpeedCmd)
|
||||
{
|
||||
case FAN_AUTO:
|
||||
fanSpeed = FUJITSU_AIRCON1_FAN_AUTO;
|
||||
break;
|
||||
case FAN_1:
|
||||
fanSpeed = FUJITSU_AIRCON1_FAN1;
|
||||
break;
|
||||
case FAN_2:
|
||||
fanSpeed = FUJITSU_AIRCON1_FAN2;
|
||||
break;
|
||||
case FAN_3:
|
||||
fanSpeed = FUJITSU_AIRCON1_FAN3;
|
||||
break;
|
||||
case FAN_4:
|
||||
fanSpeed = FUJITSU_AIRCON1_FAN4;
|
||||
break;
|
||||
}
|
||||
|
||||
if ( temperatureCmd > 15 && temperatureCmd < 31)
|
||||
{
|
||||
temperature = temperatureCmd;
|
||||
}
|
||||
|
||||
sendFujitsu(IR, operatingMode, fanSpeed, temperature);
|
||||
}
|
||||
|
||||
void FujitsuHeatpumpIR::sendFujitsu(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature)
|
||||
{
|
||||
// ON, HEAT, AUTO FAN, +24 degrees
|
||||
byte FujitsuTemplate[] = { 0x14, 0x63, 0x00, 0x10, 0x10, 0xFE, 0x09, 0x30, 0x80, 0x04, 0x00, 0x00, 0x00, 0x00, 0x20, 0x00 };
|
||||
// 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
|
||||
|
||||
static const prog_uint8_t OFF_msg[] PROGMEM = { 0x14, 0x63, 0x00, 0x10, 0x10, 0x02, 0xFD };
|
||||
byte checksum = 0x00;
|
||||
|
||||
// Set the operatingmode on the template message
|
||||
FujitsuTemplate[9] = operatingMode;
|
||||
|
||||
// Set the temperature on the template message
|
||||
FujitsuTemplate[8] = (temperature - 16) << 4;
|
||||
|
||||
// Set the fan speed on the template message
|
||||
FujitsuTemplate[10] = fanSpeed;
|
||||
|
||||
// Calculate the checksum
|
||||
for (int i=0; i<15; i++) {
|
||||
checksum += FujitsuTemplate[i];
|
||||
}
|
||||
|
||||
FujitsuTemplate[15] = (byte)(0x9E - checksum);
|
||||
|
||||
// 40 kHz PWM frequency
|
||||
IR.setFrequency(40);
|
||||
|
||||
// Header
|
||||
IR.mark(FUJITSU_AIRCON1_HDR_MARK);
|
||||
IR.space(FUJITSU_AIRCON1_HDR_SPACE);
|
||||
|
||||
if (operatingMode == FUJITSU_AIRCON1_MODE_OFF) {
|
||||
// OFF
|
||||
for (int i=0; i<sizeof(OFF_msg); i++) {
|
||||
IR.sendIRByte(OFF_msg[i], FUJITSU_AIRCON1_BIT_MARK, FUJITSU_AIRCON1_ZERO_SPACE, FUJITSU_AIRCON1_ONE_SPACE);
|
||||
}
|
||||
} else {
|
||||
// Data
|
||||
for (int i=0; i<sizeof(FujitsuTemplate); i++) {
|
||||
IR.sendIRByte(FujitsuTemplate[i], FUJITSU_AIRCON1_BIT_MARK, FUJITSU_AIRCON1_ZERO_SPACE, FUJITSU_AIRCON1_ONE_SPACE);
|
||||
}
|
||||
}
|
||||
|
||||
// End mark
|
||||
IR.mark(FUJITSU_AIRCON1_BIT_MARK);
|
||||
IR.space(0);
|
||||
}
|
|
@ -0,0 +1,43 @@
|
|||
/*
|
||||
Fujitsu Nocria (AWYZ14) heatpump control (remote control P/N AR-PZ2)
|
||||
*/
|
||||
#ifndef FujitsuHeatpumpIR_h
|
||||
#define FujitsuHeatpumpIR_h
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
#include "HeatpumpIR.h"
|
||||
|
||||
|
||||
// Fujitsu Nocria (AWYZ14) timing constants (remote control P/N AR-PZ2)
|
||||
#define FUJITSU_AIRCON1_HDR_MARK 3250
|
||||
#define FUJITSU_AIRCON1_HDR_SPACE 1550
|
||||
#define FUJITSU_AIRCON1_BIT_MARK 400
|
||||
#define FUJITSU_AIRCON1_ONE_SPACE 1200
|
||||
#define FUJITSU_AIRCON1_ZERO_SPACE 390
|
||||
|
||||
// Fujitsu codes
|
||||
#define FUJITSU_AIRCON1_MODE_AUTO 0x00 // Operating mode
|
||||
#define FUJITSU_AIRCON1_MODE_HEAT 0x04
|
||||
#define FUJITSU_AIRCON1_MODE_COOL 0x01
|
||||
#define FUJITSU_AIRCON1_MODE_DRY 0x02
|
||||
#define FUJITSU_AIRCON1_MODE_FAN 0x03
|
||||
#define FUJITSU_AIRCON1_MODE_OFF 0xFF // Power OFF - not real codes, but we need something...
|
||||
#define FUJITSU_AIRCON1_FAN_AUTO 0x00 // Fan speed
|
||||
#define FUJITSU_AIRCON1_FAN1 0x04
|
||||
#define FUJITSU_AIRCON1_FAN2 0x03
|
||||
#define FUJITSU_AIRCON1_FAN3 0x02
|
||||
#define FUJITSU_AIRCON1_FAN4 0x01
|
||||
|
||||
|
||||
class FujitsuHeatpumpIR : public HeatpumpIR
|
||||
{
|
||||
public:
|
||||
FujitsuHeatpumpIR();
|
||||
void send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd);
|
||||
|
||||
private:
|
||||
void sendFujitsu(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature);
|
||||
};
|
||||
|
||||
#endif
|
|
@ -0,0 +1,10 @@
|
|||
#include <Arduino.h>
|
||||
#include "HeatpumpIR.h"
|
||||
|
||||
HeatpumpIR::HeatpumpIR()
|
||||
{
|
||||
}
|
||||
|
||||
void HeatpumpIR::send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
}
|
|
@ -0,0 +1,64 @@
|
|||
/*
|
||||
The generic heatpump interface
|
||||
* Constants for modes
|
||||
* The constructor and the 'send' command interface
|
||||
*/
|
||||
|
||||
#ifndef HeatpumpIR_h
|
||||
#define HeatpumpIR_h
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
|
||||
|
||||
// Power state
|
||||
#define POWER_OFF 0
|
||||
#define POWER_ON 1
|
||||
|
||||
// Operating modes
|
||||
#define MODE_AUTO 1
|
||||
#define MODE_HEAT 2
|
||||
#define MODE_COOL 3
|
||||
#define MODE_DRY 4
|
||||
#define MODE_FAN 5
|
||||
#define MODE_MAINT 6
|
||||
|
||||
// Fan speeds. Note that some heatpumps have less than 5 fan speeds
|
||||
#define FAN_AUTO 1
|
||||
#define FAN_1 2
|
||||
#define FAN_2 3
|
||||
#define FAN_3 4
|
||||
#define FAN_4 5
|
||||
#define FAN_5 6
|
||||
|
||||
// Vertical air directions. Note that these cannot be set on all heat pumps
|
||||
#define VDIR_AUTO 0
|
||||
#define VDIR_MANUAL 0
|
||||
#define VDIR_SWING 1
|
||||
#define VDIR_UP 2
|
||||
#define VDIR_MUP 3
|
||||
#define VDIR_MIDDLE 4
|
||||
#define VDIR_MDOWN 5
|
||||
#define VDIR_DOWN 6
|
||||
|
||||
// Horizontal air directions. Note that these cannot be set on all heat pumps
|
||||
#define HDIR_AUTO 0
|
||||
#define HDIR_MANUAL 0
|
||||
#define HDIR_SWING 1
|
||||
#define HDIR_MIDDLE 2
|
||||
#define HDIR_LEFT 3
|
||||
#define HDIR_MLEFT 4
|
||||
#define HDIR_MRIGHT 5
|
||||
#define HDIR_RIGHT 6
|
||||
|
||||
|
||||
class HeatpumpIR
|
||||
{
|
||||
protected:
|
||||
HeatpumpIR(); // Cannot create generic heatpump instances
|
||||
|
||||
public:
|
||||
virtual void send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd);
|
||||
};
|
||||
|
||||
#endif
|
|
@ -0,0 +1,193 @@
|
|||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
|
||||
// Heavily based on Ken Shirriff's IRRemote library:
|
||||
// https://github.com/shirriff/Arduino-IRremote
|
||||
//
|
||||
// For PWM on Arduino, see http://playground.arduino.cc/Main/TimerPWMCheatsheet
|
||||
|
||||
IRSender::IRSender(int pin)
|
||||
{
|
||||
_pin = pin;
|
||||
}
|
||||
|
||||
// Set the PWM frequency. The selected pin determines which timer to use
|
||||
void IRSender::setFrequency(int frequency)
|
||||
{
|
||||
uint8_t pwmval8 = F_CPU / 2000 / (frequency);
|
||||
uint16_t pwmval16 = F_CPU / 2000 / (frequency);
|
||||
|
||||
pinMode(_pin, OUTPUT);
|
||||
digitalWrite(_pin, LOW); // When not sending PWM, we want it low
|
||||
|
||||
switch (_pin)
|
||||
{
|
||||
#if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__)
|
||||
// Arduino Mega
|
||||
case 9:
|
||||
// Fall-through to 10, timer2 controls both 9 and 10
|
||||
case 10:
|
||||
TCCR2A = _BV(WGM20);
|
||||
TCCR2B = _BV(WGM22) | _BV(CS20);
|
||||
OCR2A = pwmval8;
|
||||
OCR2B = pwmval8 / 3;
|
||||
break;
|
||||
case 11:
|
||||
// Fall-through to 12, timer1 controls both 11 and 12
|
||||
case 12:
|
||||
TCCR1A = _BV(WGM11);
|
||||
TCCR1B = _BV(WGM13) | _BV(CS10);
|
||||
ICR1 = pwmval16;
|
||||
OCR1A = pwmval16 / 3;
|
||||
OCR1B = pwmval16 / 3;
|
||||
break;
|
||||
case 46: // Timer 5 on Arduino Mega
|
||||
TCCR5A = _BV(WGM51);
|
||||
TCCR5B = _BV(WGM53) | _BV(CS50);
|
||||
ICR5 = pwmval;
|
||||
OCR5A = pwmval / 3;
|
||||
#else
|
||||
// Arduino Duemilanove etc
|
||||
case 3:
|
||||
// Fall-through to 11, timer2 controls both 3 and 11
|
||||
case 11:
|
||||
TCCR2A = _BV(WGM20);
|
||||
TCCR2B = _BV(WGM22) | _BV(CS20);
|
||||
OCR2A = pwmval8;
|
||||
OCR2B = pwmval8 / 3;
|
||||
break;
|
||||
case 9:
|
||||
// Fall-through to 10, timer1 controls both 9 and 10
|
||||
case 10:
|
||||
TCCR1A = _BV(WGM11);
|
||||
TCCR1B = _BV(WGM13) | _BV(CS10);
|
||||
ICR1 = pwmval16;
|
||||
OCR1A = pwmval16 / 3;
|
||||
OCR1B = pwmval16 / 3;
|
||||
break;
|
||||
#endif
|
||||
}
|
||||
}
|
||||
|
||||
// Send a byte (8 bits) over IR
|
||||
void IRSender::sendIRByte(byte sendByte, int bitMarkLength, int zeroSpaceLength, int oneSpaceLength)
|
||||
{
|
||||
for (int i=0; i<8 ; i++)
|
||||
{
|
||||
if (sendByte & 0x01)
|
||||
{
|
||||
mark(bitMarkLength);
|
||||
space(oneSpaceLength);
|
||||
}
|
||||
else
|
||||
{
|
||||
mark(bitMarkLength);
|
||||
space(zeroSpaceLength);
|
||||
}
|
||||
|
||||
sendByte >>= 1;
|
||||
}
|
||||
}
|
||||
|
||||
// The Carrier IR protocol has the bits in a reverse order (compared to the other heatpumps)
|
||||
// See http://www.nrtm.org/index.php/2013/07/25/reverse-bits-in-a-byte/
|
||||
byte IRSender::bitReverse(byte x)
|
||||
{
|
||||
// 01010101 | 10101010
|
||||
x = ((x >> 1) & 0x55) | ((x << 1) & 0xaa);
|
||||
// 00110011 | 11001100
|
||||
x = ((x >> 2) & 0x33) | ((x << 2) & 0xcc);
|
||||
// 00001111 | 11110000
|
||||
x = ((x >> 4) & 0x0f) | ((x << 4) & 0xf0);
|
||||
return x;
|
||||
}
|
||||
|
||||
// Send an IR 'mark' symbol, i.e. transmitter ON
|
||||
void IRSender::mark(int markLength)
|
||||
{
|
||||
switch (_pin)
|
||||
{
|
||||
#if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__)
|
||||
// Arduino Mega
|
||||
case 9:
|
||||
(TCCR2A |= _BV(COM2B1)); // Enable pin 3 PWM output
|
||||
break;
|
||||
case 11:
|
||||
(TCCR1A |= _BV(COM1A1)); // Enable pin 9 PWM output
|
||||
break;
|
||||
case 12:
|
||||
(TCCR1A |= _BV(COM1B1)); // Enable pin 10 PWM output
|
||||
break;
|
||||
case 10:
|
||||
(TCCR2A |= _BV(COM2A1)); // Enable pin 11 PWM output
|
||||
break;
|
||||
case 46:
|
||||
(TCCR5A |= _BV(COM5A1)); // Enable pin 46 PWM output on Arduino Mega
|
||||
break;
|
||||
#else
|
||||
// Arduino Duemilanove etc
|
||||
case 3:
|
||||
(TCCR2A |= _BV(COM2B1)); // Enable pin 3 PWM output
|
||||
break;
|
||||
case 9:
|
||||
(TCCR1A |= _BV(COM1A1)); // Enable pin 9 PWM output
|
||||
break;
|
||||
case 10:
|
||||
(TCCR1A |= _BV(COM1B1)); // Enable pin 10 PWM output
|
||||
break;
|
||||
case 11:
|
||||
(TCCR2A |= _BV(COM2A1)); // Enable pin 11 PWM output
|
||||
break;
|
||||
#endif
|
||||
}
|
||||
|
||||
delayMicroseconds(markLength);
|
||||
}
|
||||
|
||||
// Send an IR 'space' symbol, i.e. transmitter OFF
|
||||
void IRSender::space(int spaceLength)
|
||||
{
|
||||
switch (_pin)
|
||||
{
|
||||
#if defined(__AVR_ATmega1280__) || defined(__AVR_ATmega2560__)
|
||||
// Arduino Mega
|
||||
case 9:
|
||||
(TCCR2A &= ~(_BV(COM2B1))); // Disable pin 3 PWM output
|
||||
break;
|
||||
case 11:
|
||||
(TCCR1A &= ~(_BV(COM1A1))); // Disable pin 9 PWM output
|
||||
break;
|
||||
case 12:
|
||||
(TCCR1A &= ~(_BV(COM1B1))); // Disable pin 10 PWM output
|
||||
break;
|
||||
case 10:
|
||||
(TCCR2A &= ~(_BV(COM2A1))); // Disable pin 11 PWM output
|
||||
break;
|
||||
case 46:
|
||||
(TCCR5A &= ~(_BV(COM5A1))); // Disable pin 46 PWM output on Arduino Mega
|
||||
#else
|
||||
// Arduino Duemilanove etc
|
||||
case 3:
|
||||
(TCCR2A &= ~(_BV(COM2B1))); // Disable pin 3 PWM output
|
||||
break;
|
||||
case 9:
|
||||
(TCCR1A &= ~(_BV(COM1A1))); // Disable pin 9 PWM output
|
||||
break;
|
||||
case 10:
|
||||
(TCCR1A &= ~(_BV(COM1B1))); // Disable pin 10 PWM output
|
||||
break;
|
||||
case 11:
|
||||
(TCCR2A &= ~(_BV(COM2A1))); // Disable pin 11 PWM output
|
||||
break;
|
||||
#endif
|
||||
}
|
||||
|
||||
// Mitsubishi heatpump uses > 16383us spaces, and delayMicroseconds only works up to 2^14 - 1 us
|
||||
// Use the less accurate milliseconds delay for longer delays
|
||||
|
||||
if (spaceLength < 16383) {
|
||||
delayMicroseconds(spaceLength);
|
||||
} else {
|
||||
delay(spaceLength/1000);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,23 @@
|
|||
/*
|
||||
Class to send IR signals using the Arduino PWM
|
||||
*/
|
||||
#ifndef IRSender_h
|
||||
#define IRSender_h
|
||||
|
||||
#include <Arduino.h>
|
||||
|
||||
class IRSender
|
||||
{
|
||||
public:
|
||||
IRSender(int pin);
|
||||
void setFrequency(int frequency);
|
||||
void sendIRByte(byte sendByte, int bitMarkLength, int zeroSpaceLength, int oneSpaceLength);
|
||||
byte bitReverse(byte x);
|
||||
void space(int spaceLength);
|
||||
void mark(int markLength);
|
||||
|
||||
private:
|
||||
int _pin;
|
||||
};
|
||||
|
||||
#endif
|
|
@ -0,0 +1,339 @@
|
|||
GNU GENERAL PUBLIC LICENSE
|
||||
Version 2, June 1991
|
||||
|
||||
Copyright (C) 1989, 1991 Free Software Foundation, Inc., <http://fsf.org/>
|
||||
51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
|
||||
Everyone is permitted to copy and distribute verbatim copies
|
||||
of this license document, but changing it is not allowed.
|
||||
|
||||
Preamble
|
||||
|
||||
The licenses for most software are designed to take away your
|
||||
freedom to share and change it. By contrast, the GNU General Public
|
||||
License is intended to guarantee your freedom to share and change free
|
||||
software--to make sure the software is free for all its users. This
|
||||
General Public License applies to most of the Free Software
|
||||
Foundation's software and to any other program whose authors commit to
|
||||
using it. (Some other Free Software Foundation software is covered by
|
||||
the GNU Lesser General Public License instead.) You can apply it to
|
||||
your programs, too.
|
||||
|
||||
When we speak of free software, we are referring to freedom, not
|
||||
price. Our General Public Licenses are designed to make sure that you
|
||||
have the freedom to distribute copies of free software (and charge for
|
||||
this service if you wish), that you receive source code or can get it
|
||||
if you want it, that you can change the software or use pieces of it
|
||||
in new free programs; and that you know you can do these things.
|
||||
|
||||
To protect your rights, we need to make restrictions that forbid
|
||||
anyone to deny you these rights or to ask you to surrender the rights.
|
||||
These restrictions translate to certain responsibilities for you if you
|
||||
distribute copies of the software, or if you modify it.
|
||||
|
||||
For example, if you distribute copies of such a program, whether
|
||||
gratis or for a fee, you must give the recipients all the rights that
|
||||
you have. You must make sure that they, too, receive or can get the
|
||||
source code. And you must show them these terms so they know their
|
||||
rights.
|
||||
|
||||
We protect your rights with two steps: (1) copyright the software, and
|
||||
(2) offer you this license which gives you legal permission to copy,
|
||||
distribute and/or modify the software.
|
||||
|
||||
Also, for each author's protection and ours, we want to make certain
|
||||
that everyone understands that there is no warranty for this free
|
||||
software. If the software is modified by someone else and passed on, we
|
||||
want its recipients to know that what they have is not the original, so
|
||||
that any problems introduced by others will not reflect on the original
|
||||
authors' reputations.
|
||||
|
||||
Finally, any free program is threatened constantly by software
|
||||
patents. We wish to avoid the danger that redistributors of a free
|
||||
program will individually obtain patent licenses, in effect making the
|
||||
program proprietary. To prevent this, we have made it clear that any
|
||||
patent must be licensed for everyone's free use or not licensed at all.
|
||||
|
||||
The precise terms and conditions for copying, distribution and
|
||||
modification follow.
|
||||
|
||||
GNU GENERAL PUBLIC LICENSE
|
||||
TERMS AND CONDITIONS FOR COPYING, DISTRIBUTION AND MODIFICATION
|
||||
|
||||
0. This License applies to any program or other work which contains
|
||||
a notice placed by the copyright holder saying it may be distributed
|
||||
under the terms of this General Public License. The "Program", below,
|
||||
refers to any such program or work, and a "work based on the Program"
|
||||
means either the Program or any derivative work under copyright law:
|
||||
that is to say, a work containing the Program or a portion of it,
|
||||
either verbatim or with modifications and/or translated into another
|
||||
language. (Hereinafter, translation is included without limitation in
|
||||
the term "modification".) Each licensee is addressed as "you".
|
||||
|
||||
Activities other than copying, distribution and modification are not
|
||||
covered by this License; they are outside its scope. The act of
|
||||
running the Program is not restricted, and the output from the Program
|
||||
is covered only if its contents constitute a work based on the
|
||||
Program (independent of having been made by running the Program).
|
||||
Whether that is true depends on what the Program does.
|
||||
|
||||
1. You may copy and distribute verbatim copies of the Program's
|
||||
source code as you receive it, in any medium, provided that you
|
||||
conspicuously and appropriately publish on each copy an appropriate
|
||||
copyright notice and disclaimer of warranty; keep intact all the
|
||||
notices that refer to this License and to the absence of any warranty;
|
||||
and give any other recipients of the Program a copy of this License
|
||||
along with the Program.
|
||||
|
||||
You may charge a fee for the physical act of transferring a copy, and
|
||||
you may at your option offer warranty protection in exchange for a fee.
|
||||
|
||||
2. You may modify your copy or copies of the Program or any portion
|
||||
of it, thus forming a work based on the Program, and copy and
|
||||
distribute such modifications or work under the terms of Section 1
|
||||
above, provided that you also meet all of these conditions:
|
||||
|
||||
a) You must cause the modified files to carry prominent notices
|
||||
stating that you changed the files and the date of any change.
|
||||
|
||||
b) You must cause any work that you distribute or publish, that in
|
||||
whole or in part contains or is derived from the Program or any
|
||||
part thereof, to be licensed as a whole at no charge to all third
|
||||
parties under the terms of this License.
|
||||
|
||||
c) If the modified program normally reads commands interactively
|
||||
when run, you must cause it, when started running for such
|
||||
interactive use in the most ordinary way, to print or display an
|
||||
announcement including an appropriate copyright notice and a
|
||||
notice that there is no warranty (or else, saying that you provide
|
||||
a warranty) and that users may redistribute the program under
|
||||
these conditions, and telling the user how to view a copy of this
|
||||
License. (Exception: if the Program itself is interactive but
|
||||
does not normally print such an announcement, your work based on
|
||||
the Program is not required to print an announcement.)
|
||||
|
||||
These requirements apply to the modified work as a whole. If
|
||||
identifiable sections of that work are not derived from the Program,
|
||||
and can be reasonably considered independent and separate works in
|
||||
themselves, then this License, and its terms, do not apply to those
|
||||
sections when you distribute them as separate works. But when you
|
||||
distribute the same sections as part of a whole which is a work based
|
||||
on the Program, the distribution of the whole must be on the terms of
|
||||
this License, whose permissions for other licensees extend to the
|
||||
entire whole, and thus to each and every part regardless of who wrote it.
|
||||
|
||||
Thus, it is not the intent of this section to claim rights or contest
|
||||
your rights to work written entirely by you; rather, the intent is to
|
||||
exercise the right to control the distribution of derivative or
|
||||
collective works based on the Program.
|
||||
|
||||
In addition, mere aggregation of another work not based on the Program
|
||||
with the Program (or with a work based on the Program) on a volume of
|
||||
a storage or distribution medium does not bring the other work under
|
||||
the scope of this License.
|
||||
|
||||
3. You may copy and distribute the Program (or a work based on it,
|
||||
under Section 2) in object code or executable form under the terms of
|
||||
Sections 1 and 2 above provided that you also do one of the following:
|
||||
|
||||
a) Accompany it with the complete corresponding machine-readable
|
||||
source code, which must be distributed under the terms of Sections
|
||||
1 and 2 above on a medium customarily used for software interchange; or,
|
||||
|
||||
b) Accompany it with a written offer, valid for at least three
|
||||
years, to give any third party, for a charge no more than your
|
||||
cost of physically performing source distribution, a complete
|
||||
machine-readable copy of the corresponding source code, to be
|
||||
distributed under the terms of Sections 1 and 2 above on a medium
|
||||
customarily used for software interchange; or,
|
||||
|
||||
c) Accompany it with the information you received as to the offer
|
||||
to distribute corresponding source code. (This alternative is
|
||||
allowed only for noncommercial distribution and only if you
|
||||
received the program in object code or executable form with such
|
||||
an offer, in accord with Subsection b above.)
|
||||
|
||||
The source code for a work means the preferred form of the work for
|
||||
making modifications to it. For an executable work, complete source
|
||||
code means all the source code for all modules it contains, plus any
|
||||
associated interface definition files, plus the scripts used to
|
||||
control compilation and installation of the executable. However, as a
|
||||
special exception, the source code distributed need not include
|
||||
anything that is normally distributed (in either source or binary
|
||||
form) with the major components (compiler, kernel, and so on) of the
|
||||
operating system on which the executable runs, unless that component
|
||||
itself accompanies the executable.
|
||||
|
||||
If distribution of executable or object code is made by offering
|
||||
access to copy from a designated place, then offering equivalent
|
||||
access to copy the source code from the same place counts as
|
||||
distribution of the source code, even though third parties are not
|
||||
compelled to copy the source along with the object code.
|
||||
|
||||
4. You may not copy, modify, sublicense, or distribute the Program
|
||||
except as expressly provided under this License. Any attempt
|
||||
otherwise to copy, modify, sublicense or distribute the Program is
|
||||
void, and will automatically terminate your rights under this License.
|
||||
However, parties who have received copies, or rights, from you under
|
||||
this License will not have their licenses terminated so long as such
|
||||
parties remain in full compliance.
|
||||
|
||||
5. You are not required to accept this License, since you have not
|
||||
signed it. However, nothing else grants you permission to modify or
|
||||
distribute the Program or its derivative works. These actions are
|
||||
prohibited by law if you do not accept this License. Therefore, by
|
||||
modifying or distributing the Program (or any work based on the
|
||||
Program), you indicate your acceptance of this License to do so, and
|
||||
all its terms and conditions for copying, distributing or modifying
|
||||
the Program or works based on it.
|
||||
|
||||
6. Each time you redistribute the Program (or any work based on the
|
||||
Program), the recipient automatically receives a license from the
|
||||
original licensor to copy, distribute or modify the Program subject to
|
||||
these terms and conditions. You may not impose any further
|
||||
restrictions on the recipients' exercise of the rights granted herein.
|
||||
You are not responsible for enforcing compliance by third parties to
|
||||
this License.
|
||||
|
||||
7. If, as a consequence of a court judgment or allegation of patent
|
||||
infringement or for any other reason (not limited to patent issues),
|
||||
conditions are imposed on you (whether by court order, agreement or
|
||||
otherwise) that contradict the conditions of this License, they do not
|
||||
excuse you from the conditions of this License. If you cannot
|
||||
distribute so as to satisfy simultaneously your obligations under this
|
||||
License and any other pertinent obligations, then as a consequence you
|
||||
may not distribute the Program at all. For example, if a patent
|
||||
license would not permit royalty-free redistribution of the Program by
|
||||
all those who receive copies directly or indirectly through you, then
|
||||
the only way you could satisfy both it and this License would be to
|
||||
refrain entirely from distribution of the Program.
|
||||
|
||||
If any portion of this section is held invalid or unenforceable under
|
||||
any particular circumstance, the balance of the section is intended to
|
||||
apply and the section as a whole is intended to apply in other
|
||||
circumstances.
|
||||
|
||||
It is not the purpose of this section to induce you to infringe any
|
||||
patents or other property right claims or to contest validity of any
|
||||
such claims; this section has the sole purpose of protecting the
|
||||
integrity of the free software distribution system, which is
|
||||
implemented by public license practices. Many people have made
|
||||
generous contributions to the wide range of software distributed
|
||||
through that system in reliance on consistent application of that
|
||||
system; it is up to the author/donor to decide if he or she is willing
|
||||
to distribute software through any other system and a licensee cannot
|
||||
impose that choice.
|
||||
|
||||
This section is intended to make thoroughly clear what is believed to
|
||||
be a consequence of the rest of this License.
|
||||
|
||||
8. If the distribution and/or use of the Program is restricted in
|
||||
certain countries either by patents or by copyrighted interfaces, the
|
||||
original copyright holder who places the Program under this License
|
||||
may add an explicit geographical distribution limitation excluding
|
||||
those countries, so that distribution is permitted only in or among
|
||||
countries not thus excluded. In such case, this License incorporates
|
||||
the limitation as if written in the body of this License.
|
||||
|
||||
9. The Free Software Foundation may publish revised and/or new versions
|
||||
of the General Public License from time to time. Such new versions will
|
||||
be similar in spirit to the present version, but may differ in detail to
|
||||
address new problems or concerns.
|
||||
|
||||
Each version is given a distinguishing version number. If the Program
|
||||
specifies a version number of this License which applies to it and "any
|
||||
later version", you have the option of following the terms and conditions
|
||||
either of that version or of any later version published by the Free
|
||||
Software Foundation. If the Program does not specify a version number of
|
||||
this License, you may choose any version ever published by the Free Software
|
||||
Foundation.
|
||||
|
||||
10. If you wish to incorporate parts of the Program into other free
|
||||
programs whose distribution conditions are different, write to the author
|
||||
to ask for permission. For software which is copyrighted by the Free
|
||||
Software Foundation, write to the Free Software Foundation; we sometimes
|
||||
make exceptions for this. Our decision will be guided by the two goals
|
||||
of preserving the free status of all derivatives of our free software and
|
||||
of promoting the sharing and reuse of software generally.
|
||||
|
||||
NO WARRANTY
|
||||
|
||||
11. BECAUSE THE PROGRAM IS LICENSED FREE OF CHARGE, THERE IS NO WARRANTY
|
||||
FOR THE PROGRAM, TO THE EXTENT PERMITTED BY APPLICABLE LAW. EXCEPT WHEN
|
||||
OTHERWISE STATED IN WRITING THE COPYRIGHT HOLDERS AND/OR OTHER PARTIES
|
||||
PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY OF ANY KIND, EITHER EXPRESSED
|
||||
OR IMPLIED, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF
|
||||
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE. THE ENTIRE RISK AS
|
||||
TO THE QUALITY AND PERFORMANCE OF THE PROGRAM IS WITH YOU. SHOULD THE
|
||||
PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF ALL NECESSARY SERVICING,
|
||||
REPAIR OR CORRECTION.
|
||||
|
||||
12. IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING
|
||||
WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MAY MODIFY AND/OR
|
||||
REDISTRIBUTE THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES,
|
||||
INCLUDING ANY GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING
|
||||
OUT OF THE USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED
|
||||
TO LOSS OF DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY
|
||||
YOU OR THIRD PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER
|
||||
PROGRAMS), EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE
|
||||
POSSIBILITY OF SUCH DAMAGES.
|
||||
|
||||
END OF TERMS AND CONDITIONS
|
||||
|
||||
How to Apply These Terms to Your New Programs
|
||||
|
||||
If you develop a new program, and you want it to be of the greatest
|
||||
possible use to the public, the best way to achieve this is to make it
|
||||
free software which everyone can redistribute and change under these terms.
|
||||
|
||||
To do so, attach the following notices to the program. It is safest
|
||||
to attach them to the start of each source file to most effectively
|
||||
convey the exclusion of warranty; and each file should have at least
|
||||
the "copyright" line and a pointer to where the full notice is found.
|
||||
|
||||
{description}
|
||||
Copyright (C) {year} {fullname}
|
||||
|
||||
This program is free software; you can redistribute it and/or modify
|
||||
it under the terms of the GNU General Public License as published by
|
||||
the Free Software Foundation; either version 2 of the License, or
|
||||
(at your option) any later version.
|
||||
|
||||
This program is distributed in the hope that it will be useful,
|
||||
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
GNU General Public License for more details.
|
||||
|
||||
You should have received a copy of the GNU General Public License along
|
||||
with this program; if not, write to the Free Software Foundation, Inc.,
|
||||
51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
||||
|
||||
Also add information on how to contact you by electronic and paper mail.
|
||||
|
||||
If the program is interactive, make it output a short notice like this
|
||||
when it starts in an interactive mode:
|
||||
|
||||
Gnomovision version 69, Copyright (C) year name of author
|
||||
Gnomovision comes with ABSOLUTELY NO WARRANTY; for details type `show w'.
|
||||
This is free software, and you are welcome to redistribute it
|
||||
under certain conditions; type `show c' for details.
|
||||
|
||||
The hypothetical commands `show w' and `show c' should show the appropriate
|
||||
parts of the General Public License. Of course, the commands you use may
|
||||
be called something other than `show w' and `show c'; they could even be
|
||||
mouse-clicks or menu items--whatever suits your program.
|
||||
|
||||
You should also get your employer (if you work as a programmer) or your
|
||||
school, if any, to sign a "copyright disclaimer" for the program, if
|
||||
necessary. Here is a sample; alter the names:
|
||||
|
||||
Yoyodyne, Inc., hereby disclaims all copyright interest in the program
|
||||
`Gnomovision' (which makes passes at compilers) written by James Hacker.
|
||||
|
||||
{signature of Ty Coon}, 1 April 1989
|
||||
Ty Coon, President of Vice
|
||||
|
||||
This General Public License does not permit incorporating your program into
|
||||
proprietary programs. If your program is a subroutine library, you may
|
||||
consider it more useful to permit linking proprietary applications with the
|
||||
library. If this is what you want to do, use the GNU Lesser General
|
||||
Public License instead of this License.
|
|
@ -0,0 +1,141 @@
|
|||
#include <Arduino.h>
|
||||
#include "MideaHeatpumpIR.h"
|
||||
|
||||
MideaHeatpumpIR::MideaHeatpumpIR()
|
||||
{
|
||||
}
|
||||
|
||||
void MideaHeatpumpIR::send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
// Sensible defaults for the heat pump mode
|
||||
byte operatingMode = MIDEA_AIRCON1_MODE_HEAT;
|
||||
byte fanSpeed = MIDEA_AIRCON1_FAN_AUTO;
|
||||
byte temperature = 23;
|
||||
|
||||
switch (powerModeCmd)
|
||||
{
|
||||
case 0:
|
||||
// OFF is a special case
|
||||
operatingMode = MIDEA_AIRCON1_MODE_OFF;
|
||||
sendMidea(IR, operatingMode, fanSpeed, temperature);
|
||||
return;
|
||||
}
|
||||
|
||||
switch (operatingModeCmd)
|
||||
{
|
||||
case MODE_AUTO:
|
||||
operatingMode = MIDEA_AIRCON1_MODE_AUTO;
|
||||
break;
|
||||
case MODE_HEAT:
|
||||
operatingMode = MIDEA_AIRCON1_MODE_HEAT;
|
||||
break;
|
||||
case MODE_COOL:
|
||||
operatingMode = MIDEA_AIRCON1_MODE_COOL;
|
||||
break;
|
||||
case MODE_DRY:
|
||||
operatingMode = MIDEA_AIRCON1_MODE_DRY;
|
||||
break;
|
||||
case MODE_FAN:
|
||||
operatingMode = MIDEA_AIRCON1_MODE_FAN;
|
||||
break;
|
||||
case MODE_MAINT:
|
||||
// Maintenance mode ('FP' on the remote) is a special mode on Midea
|
||||
operatingMode = MIDEA_AIRCON1_MODE_FP;
|
||||
sendMidea(IR, operatingMode, fanSpeed, temperature);
|
||||
return;
|
||||
}
|
||||
|
||||
switch (fanSpeedCmd)
|
||||
{
|
||||
case FAN_AUTO:
|
||||
fanSpeed = MIDEA_AIRCON1_FAN_AUTO;
|
||||
break;
|
||||
case FAN_1:
|
||||
fanSpeed = MIDEA_AIRCON1_FAN1;
|
||||
break;
|
||||
case FAN_2:
|
||||
fanSpeed = MIDEA_AIRCON1_FAN2;
|
||||
break;
|
||||
case FAN_3:
|
||||
fanSpeed = MIDEA_AIRCON1_FAN3;
|
||||
break;
|
||||
}
|
||||
|
||||
if ( temperatureCmd > 15 && temperatureCmd < 31)
|
||||
{
|
||||
temperature = temperatureCmd;
|
||||
}
|
||||
|
||||
sendMidea(IR, operatingMode, fanSpeed, temperature);
|
||||
}
|
||||
|
||||
// Send the Midea code
|
||||
void MideaHeatpumpIR::sendMidea(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature)
|
||||
{
|
||||
byte sendBuffer[3] = { 0x4D, 0x00, 0x00 }; // First byte is always 0x4D
|
||||
|
||||
static const prog_uint8_t temperatures[] PROGMEM = {0, 8, 12, 4, 6, 14, 10, 2, 3, 11, 9, 1, 5, 13 };
|
||||
|
||||
static const prog_uint8_t OffMsg[] PROGMEM = {0x4D, 0xDE, 0x07 };
|
||||
static const prog_uint8_t FPMsg[] PROGMEM = {0xAD, 0xAF, 0xB5 };
|
||||
|
||||
if (operatingMode == MIDEA_AIRCON1_MODE_OFF)
|
||||
{
|
||||
memcpy_P(sendBuffer, OffMsg, sizeof(sendBuffer));
|
||||
}
|
||||
else if (operatingMode == MIDEA_AIRCON1_MODE_FP)
|
||||
{
|
||||
memcpy_P(sendBuffer, FPMsg, sizeof(sendBuffer));
|
||||
}
|
||||
else
|
||||
{
|
||||
sendBuffer[1] = ~fanSpeed;
|
||||
|
||||
if ( operatingMode == MIDEA_AIRCON1_MODE_FAN )
|
||||
{
|
||||
sendBuffer[2] = MIDEA_AIRCON1_MODE_DRY | 0x07;
|
||||
}
|
||||
else
|
||||
{
|
||||
sendBuffer[2] = operatingMode | temperatures[temperature-17];
|
||||
}
|
||||
}
|
||||
|
||||
// Send the code
|
||||
sendMidearaw(IR, sendBuffer);
|
||||
}
|
||||
|
||||
// Send the Midea raw code
|
||||
void MideaHeatpumpIR::sendMidearaw(IRSender& IR, byte sendBuffer[])
|
||||
{
|
||||
// 40 kHz PWM frequency
|
||||
IR.setFrequency(40);
|
||||
|
||||
// Header
|
||||
IR.mark(MIDEA_AIRCON1_HDR_MARK);
|
||||
IR.space(MIDEA_AIRCON1_HDR_SPACE);
|
||||
|
||||
// Six bytes, every second byte is a bitwise not of the previous byte
|
||||
for (int i=0; i<3; i++) {
|
||||
IR.sendIRByte(sendBuffer[i], MIDEA_AIRCON1_BIT_MARK, MIDEA_AIRCON1_ZERO_SPACE, MIDEA_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(~sendBuffer[i], MIDEA_AIRCON1_BIT_MARK, MIDEA_AIRCON1_ZERO_SPACE, MIDEA_AIRCON1_ONE_SPACE);
|
||||
}
|
||||
|
||||
// Pause
|
||||
IR.mark(MIDEA_AIRCON1_BIT_MARK);
|
||||
IR.space(MIDEA_AIRCON1_MSG_SPACE);
|
||||
|
||||
// Header, two last bytes repeated
|
||||
IR.mark(MIDEA_AIRCON1_HDR_MARK);
|
||||
IR.space(MIDEA_AIRCON1_HDR_SPACE);
|
||||
|
||||
// Six bytes, every second byte is a bitwise not of the previous byte
|
||||
for (int i=0; i<3; i++) {
|
||||
IR.sendIRByte(sendBuffer[i], MIDEA_AIRCON1_BIT_MARK, MIDEA_AIRCON1_ZERO_SPACE, MIDEA_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(~sendBuffer[i], MIDEA_AIRCON1_BIT_MARK, MIDEA_AIRCON1_ZERO_SPACE, MIDEA_AIRCON1_ONE_SPACE);
|
||||
}
|
||||
|
||||
// End mark
|
||||
IR.mark(MIDEA_AIRCON1_BIT_MARK);
|
||||
IR.space(0);
|
||||
}
|
|
@ -0,0 +1,46 @@
|
|||
/*
|
||||
Midea MSR1-12HRN1-QC2 + MOA1-12HN1-QC2 heatpump control (remote control P/N RG51M1/E)
|
||||
This heatpump is sold as 'Ultimate Pro Plus 13FP' in Finland, by www.ultimatemarket.com
|
||||
*/
|
||||
#ifndef MideaHeatpumpIR_h
|
||||
#define MideaHeatpumpIR_h
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
#include "HeatpumpIR.h"
|
||||
|
||||
// Midea timing constants, Midea MSR1-12HRN1-QC2 + MOA1-12HN1-QC2, sold as Ultimate Pro Plus Basic 13FP in Finland (remote control P/N RG51M1/E)
|
||||
#define MIDEA_AIRCON1_HDR_MARK 4350
|
||||
#define MIDEA_AIRCON1_HDR_SPACE 4230
|
||||
#define MIDEA_AIRCON1_BIT_MARK 520
|
||||
#define MIDEA_AIRCON1_ONE_SPACE 1650
|
||||
#define MIDEA_AIRCON1_ZERO_SPACE 550
|
||||
#define MIDEA_AIRCON1_MSG_SPACE 5100
|
||||
|
||||
// MIDEA codes
|
||||
#define MIDEA_AIRCON1_MODE_AUTO 0x10 // Operating mode
|
||||
#define MIDEA_AIRCON1_MODE_HEAT 0x30
|
||||
#define MIDEA_AIRCON1_MODE_COOL 0x00
|
||||
#define MIDEA_AIRCON1_MODE_DRY 0x20
|
||||
#define MIDEA_AIRCON1_MODE_FAN 0x60
|
||||
#define MIDEA_AIRCON1_MODE_FP 0x70 // Not a real mode...
|
||||
#define MIDEA_AIRCON1_MODE_OFF 0xFE // Power OFF - not real codes, but we need something...
|
||||
#define MIDEA_AIRCON1_MODE_ON 0xFF // Power ON
|
||||
#define MIDEA_AIRCON1_FAN_AUTO 0x02 // Fan speed
|
||||
#define MIDEA_AIRCON1_FAN1 0x06
|
||||
#define MIDEA_AIRCON1_FAN2 0x05
|
||||
#define MIDEA_AIRCON1_FAN3 0x03
|
||||
|
||||
|
||||
class MideaHeatpumpIR : public HeatpumpIR
|
||||
{
|
||||
public:
|
||||
MideaHeatpumpIR();
|
||||
void send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd);
|
||||
|
||||
private:
|
||||
void sendMidea(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature);
|
||||
void sendMidearaw(IRSender& IR, byte sendBuffer[]);
|
||||
};
|
||||
|
||||
#endif
|
|
@ -0,0 +1,118 @@
|
|||
#include <Arduino.h>
|
||||
#include "MitsubishiHeatpumpIR.h"
|
||||
|
||||
MitsubishiHeatpumpIR::MitsubishiHeatpumpIR()
|
||||
{
|
||||
}
|
||||
|
||||
void MitsubishiHeatpumpIR::send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
// Sensible defaults for the heat pump mode
|
||||
|
||||
byte powerMode = MITSUBISHI_AIRCON1_MODE_ON;
|
||||
byte operatingMode = MITSUBISHI_AIRCON1_MODE_HEAT;
|
||||
byte fanSpeed = MITSUBISHI_AIRCON1_FAN_AUTO;
|
||||
byte temperature = 23;
|
||||
|
||||
if (powerModeCmd == 0)
|
||||
{
|
||||
powerMode = MITSUBISHI_AIRCON1_MODE_OFF;
|
||||
}
|
||||
|
||||
switch (operatingModeCmd)
|
||||
{
|
||||
case MODE_AUTO:
|
||||
operatingMode = MITSUBISHI_AIRCON1_MODE_AUTO;
|
||||
break;
|
||||
case MODE_HEAT:
|
||||
operatingMode = MITSUBISHI_AIRCON1_MODE_HEAT;
|
||||
break;
|
||||
case MODE_COOL:
|
||||
operatingMode = MITSUBISHI_AIRCON1_MODE_COOL;
|
||||
break;
|
||||
case MODE_DRY:
|
||||
operatingMode = MITSUBISHI_AIRCON1_MODE_DRY;
|
||||
break;
|
||||
case MODE_FAN:
|
||||
operatingMode = MITSUBISHI_AIRCON1_MODE_COOL;
|
||||
// Temperature needs to be set to 31 degrees for 'simulated' FAN mode
|
||||
temperatureCmd = 31;
|
||||
break;
|
||||
}
|
||||
|
||||
switch (fanSpeedCmd)
|
||||
{
|
||||
case FAN_AUTO:
|
||||
fanSpeed = MITSUBISHI_AIRCON1_FAN_AUTO;
|
||||
break;
|
||||
case FAN_1:
|
||||
fanSpeed = MITSUBISHI_AIRCON1_FAN1;
|
||||
break;
|
||||
case FAN_2:
|
||||
fanSpeed = MITSUBISHI_AIRCON1_FAN2;
|
||||
break;
|
||||
case FAN_3:
|
||||
fanSpeed = MITSUBISHI_AIRCON1_FAN3;
|
||||
break;
|
||||
case FAN_4:
|
||||
fanSpeed = MITSUBISHI_AIRCON1_FAN4;
|
||||
break;
|
||||
}
|
||||
|
||||
if ( temperatureCmd > 15 && temperatureCmd < 32)
|
||||
{
|
||||
temperature = temperatureCmd;
|
||||
}
|
||||
|
||||
sendMitsubishi(IR, powerMode, operatingMode, fanSpeed, temperature, 0, 0);
|
||||
}
|
||||
|
||||
void MitsubishiHeatpumpIR::sendMitsubishi(IRSender& IR, byte powerMode, byte operatingMode, byte fanSpeed, byte temperature, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
byte MitsubishiTemplate[] = { 0x23, 0xCB, 0x26, 0x01, 0x00, 0x20, 0x48, 0x00, 0xC0, 0x7A, 0x61, 0x00, 0x00, 0x00, 0x10, 0x40, 0x00, 0x00 };
|
||||
// 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
|
||||
|
||||
byte checksum = 0x00;
|
||||
|
||||
// Set the operatingmode on the template message
|
||||
MitsubishiTemplate[5] = powerMode;
|
||||
MitsubishiTemplate[6] = operatingMode;
|
||||
|
||||
// Set the temperature on the template message
|
||||
MitsubishiTemplate[7] = temperature - 16;
|
||||
|
||||
// Set the operatingmode on the template message
|
||||
MitsubishiTemplate[9] = fanSpeed;
|
||||
|
||||
// Calculate the checksum
|
||||
for (int i=0; i<17; i++) {
|
||||
checksum += MitsubishiTemplate[i];
|
||||
}
|
||||
|
||||
MitsubishiTemplate[17] = checksum;
|
||||
|
||||
// 40 kHz PWM frequency
|
||||
IR.setFrequency(40);
|
||||
|
||||
// The Mitsubishi data is repeated twice
|
||||
for (int j=0; j<2; j++) {
|
||||
// Header
|
||||
IR.mark(MITSUBISHI_AIRCON1_HDR_MARK);
|
||||
IR.space(MITSUBISHI_AIRCON1_HDR_SPACE);
|
||||
|
||||
// Data
|
||||
for (int i=0; i<sizeof(MitsubishiTemplate); i++) {
|
||||
IR.sendIRByte(MitsubishiTemplate[i], MITSUBISHI_AIRCON1_BIT_MARK, MITSUBISHI_AIRCON1_ZERO_SPACE, MITSUBISHI_AIRCON1_ONE_SPACE);
|
||||
}
|
||||
|
||||
// Pause between the first and the second data burst
|
||||
if (j == 0) {
|
||||
IR.mark(MITSUBISHI_AIRCON1_BIT_MARK);
|
||||
IR.space(MITSUBISHI_AIRCON1_MSG_SPACE);
|
||||
}
|
||||
}
|
||||
|
||||
// End mark
|
||||
IR.mark(MITSUBISHI_AIRCON1_BIT_MARK);
|
||||
IR.space(0);
|
||||
}
|
|
@ -0,0 +1,44 @@
|
|||
/*
|
||||
Mitsubishi MSZ FD-25 heatpump control (remote control P/N KM09D 0052376)
|
||||
*/
|
||||
#ifndef MitsubishiHeatpumpIR_h
|
||||
#define MitsubishiHeatpumpIR_h
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
#include "HeatpumpIR.h"
|
||||
|
||||
|
||||
// Mitsubishi MSZ FD-25 timing constants (remote control P/N KM09D 0052376)
|
||||
#define MITSUBISHI_AIRCON1_HDR_MARK 3500
|
||||
#define MITSUBISHI_AIRCON1_HDR_SPACE 1700
|
||||
#define MITSUBISHI_AIRCON1_BIT_MARK 430
|
||||
#define MITSUBISHI_AIRCON1_ONE_SPACE 1250
|
||||
#define MITSUBISHI_AIRCON1_ZERO_SPACE 390
|
||||
#define MITSUBISHI_AIRCON1_MSG_SPACE 17500
|
||||
|
||||
// Mitsubishi codes
|
||||
#define MITSUBISHI_AIRCON1_MODE_AUTO 0x60 // Operating mode
|
||||
#define MITSUBISHI_AIRCON1_MODE_HEAT 0x48
|
||||
#define MITSUBISHI_AIRCON1_MODE_COOL 0x58
|
||||
#define MITSUBISHI_AIRCON1_MODE_DRY 0x50
|
||||
#define MITSUBISHI_AIRCON1_MODE_OFF 0x00 // Power OFF
|
||||
#define MITSUBISHI_AIRCON1_MODE_ON 0x20 // Power ON
|
||||
#define MITSUBISHI_AIRCON1_FAN_AUTO 0xB8 // Fan speed - mixed with vertical swing...
|
||||
#define MITSUBISHI_AIRCON1_FAN1 0x79
|
||||
#define MITSUBISHI_AIRCON1_FAN2 0x7A
|
||||
#define MITSUBISHI_AIRCON1_FAN3 0x7B
|
||||
#define MITSUBISHI_AIRCON1_FAN4 0x7C
|
||||
|
||||
|
||||
class MitsubishiHeatpumpIR : public HeatpumpIR
|
||||
{
|
||||
public:
|
||||
MitsubishiHeatpumpIR();
|
||||
void send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd);
|
||||
|
||||
private:
|
||||
void sendMitsubishi(IRSender& IR, byte powerMode, byte operatingMode, byte fanSpeed, byte temperature, byte swingVCmd, byte swingHCmd);
|
||||
};
|
||||
|
||||
#endif
|
|
@ -0,0 +1,257 @@
|
|||
#include <Arduino.h>
|
||||
#include "PanasonicCKPHeatpumpIR.h"
|
||||
|
||||
|
||||
PanasonicCKPHeatpumpIR::PanasonicCKPHeatpumpIR()
|
||||
{
|
||||
}
|
||||
|
||||
// Panasonic CKP numeric values to command bytes
|
||||
void PanasonicCKPHeatpumpIR::send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
// Sensible defaults for the heat pump mode
|
||||
|
||||
byte powerMode = false;
|
||||
byte operatingMode = PANASONIC_AIRCON1_MODE_KEEP;
|
||||
byte fanSpeed = PANASONIC_AIRCON1_FAN_AUTO;
|
||||
byte temperature = 23;
|
||||
byte swingV = PANASONIC_AIRCON1_VS_UP;
|
||||
byte swingH = PANASONIC_AIRCON1_HS_SWING;
|
||||
|
||||
switch (powerModeCmd)
|
||||
{
|
||||
case POWER_ON:
|
||||
powerMode = true;
|
||||
break;
|
||||
}
|
||||
|
||||
switch (operatingModeCmd)
|
||||
{
|
||||
case MODE_AUTO:
|
||||
operatingMode |= PANASONIC_AIRCON1_MODE_AUTO;
|
||||
break;
|
||||
case MODE_HEAT:
|
||||
operatingMode |= PANASONIC_AIRCON1_MODE_HEAT;
|
||||
break;
|
||||
case MODE_COOL:
|
||||
operatingMode |= PANASONIC_AIRCON1_MODE_COOL;
|
||||
break;
|
||||
case MODE_DRY:
|
||||
operatingMode |= PANASONIC_AIRCON1_MODE_DRY;
|
||||
break;
|
||||
case MODE_FAN:
|
||||
operatingMode |= PANASONIC_AIRCON1_MODE_FAN;
|
||||
temperatureCmd = 27; // Temperature is always 27 in FAN mode
|
||||
break;
|
||||
default:
|
||||
operatingMode |= PANASONIC_AIRCON1_MODE_HEAT;
|
||||
break;
|
||||
}
|
||||
|
||||
switch (fanSpeedCmd)
|
||||
{
|
||||
case FAN_AUTO:
|
||||
fanSpeed = PANASONIC_AIRCON1_FAN_AUTO;
|
||||
break;
|
||||
case FAN_1:
|
||||
fanSpeed = PANASONIC_AIRCON1_FAN1;
|
||||
break;
|
||||
case FAN_2:
|
||||
fanSpeed = PANASONIC_AIRCON1_FAN2;
|
||||
break;
|
||||
case FAN_3:
|
||||
fanSpeed = PANASONIC_AIRCON1_FAN3;
|
||||
break;
|
||||
case FAN_4:
|
||||
fanSpeed = PANASONIC_AIRCON1_FAN4;
|
||||
break;
|
||||
case FAN_5:
|
||||
fanSpeed = PANASONIC_AIRCON1_FAN5;
|
||||
break;
|
||||
}
|
||||
|
||||
if ( temperatureCmd > 15 && temperatureCmd < 31)
|
||||
{
|
||||
temperature = temperatureCmd;
|
||||
}
|
||||
|
||||
switch (swingVCmd)
|
||||
{
|
||||
case VDIR_SWING:
|
||||
swingV = PANASONIC_AIRCON1_VS_SWING;
|
||||
break;
|
||||
case VDIR_UP:
|
||||
swingV = PANASONIC_AIRCON1_VS_UP;
|
||||
break;
|
||||
case VDIR_MUP:
|
||||
swingV = PANASONIC_AIRCON1_VS_MUP;
|
||||
break;
|
||||
case VDIR_MIDDLE:
|
||||
swingV = PANASONIC_AIRCON1_VS_MIDDLE;
|
||||
break;
|
||||
case VDIR_MDOWN:
|
||||
swingV = PANASONIC_AIRCON1_VS_MDOWN;
|
||||
break;
|
||||
case VDIR_DOWN:
|
||||
swingV = PANASONIC_AIRCON1_VS_DOWN;
|
||||
break;
|
||||
}
|
||||
|
||||
switch (swingHCmd)
|
||||
{
|
||||
case HDIR_SWING:
|
||||
swingH = PANASONIC_AIRCON1_HS_SWING;
|
||||
break;
|
||||
case HDIR_AUTO: // Well, just set it to manual
|
||||
swingH = PANASONIC_AIRCON1_HS_MANUAL;
|
||||
break;
|
||||
}
|
||||
|
||||
sendPanasonicCKP(IR, operatingMode, fanSpeed, temperature, swingV, swingH);
|
||||
delay(1000); // Sleep 1 second between the messages
|
||||
|
||||
// This will change the power state in one minute from now
|
||||
sendPanasonicCKPOnOffTimerCancel(IR, powerMode, false);
|
||||
/*
|
||||
// Send the 'timer cancel' signal 2 minutes later
|
||||
if (panasonicCancelTimer != 0)
|
||||
{
|
||||
timer.stop(panasonicCancelTimer);
|
||||
panasonicCancelTimer = 0;
|
||||
}
|
||||
|
||||
// Note that the argument to 'timer.after' has to be explicitly cast into 'long'
|
||||
panasonicCancelTimer = timer.after(2L*60L*1000L, sendPanasonicCKPCancelTimer);
|
||||
|
||||
Serial.print(F("'Timer cancel' timer ID: "));
|
||||
Serial.println(panasonicCancelTimer);
|
||||
*/
|
||||
}
|
||||
|
||||
// Send the Panasonic CKP code
|
||||
void PanasonicCKPHeatpumpIR::sendPanasonicCKP(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature, byte swingV, byte swingH)
|
||||
{
|
||||
byte sendBuffer[4];
|
||||
|
||||
// Fan speed & temperature, temperature needs to be 27 in FAN mode
|
||||
if (operatingMode == PANASONIC_AIRCON1_MODE_FAN || operatingMode == (PANASONIC_AIRCON1_MODE_FAN | PANASONIC_AIRCON1_MODE_KEEP ))
|
||||
{
|
||||
temperature = 27;
|
||||
}
|
||||
|
||||
sendBuffer[0] = fanSpeed | (temperature - 15);
|
||||
|
||||
// Power toggle & operation mode
|
||||
sendBuffer[1] = operatingMode;
|
||||
|
||||
// Swings
|
||||
sendBuffer[2] = swingV | swingH;
|
||||
|
||||
// Always 0x36
|
||||
sendBuffer[3] = 0x36;
|
||||
|
||||
// Send the code
|
||||
sendPanasonicCKPraw(IR, sendBuffer);
|
||||
}
|
||||
|
||||
// Send the Panasonic CKP raw code
|
||||
void PanasonicCKPHeatpumpIR::sendPanasonicCKPraw(IRSender& IR, byte sendBuffer[])
|
||||
{
|
||||
// 40 kHz PWM frequency
|
||||
IR.setFrequency(40);
|
||||
|
||||
// Header, two first bytes repeated
|
||||
IR.mark(PANASONIC_AIRCON1_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_HDR_SPACE);
|
||||
|
||||
for (int i=0; i<2; i++) {
|
||||
IR.sendIRByte(sendBuffer[0], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[0], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[1], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[1], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
|
||||
IR.mark(PANASONIC_AIRCON1_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_HDR_SPACE);
|
||||
}
|
||||
|
||||
// Pause
|
||||
|
||||
IR.mark(PANASONIC_AIRCON1_BIT_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_MSG_SPACE);
|
||||
|
||||
// Header, two last bytes repeated
|
||||
|
||||
IR.mark(PANASONIC_AIRCON1_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_HDR_SPACE);
|
||||
|
||||
for (int i=0; i<2; i++) {
|
||||
IR.sendIRByte(sendBuffer[2], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[2], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[3], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[3], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
|
||||
IR.mark(PANASONIC_AIRCON1_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_HDR_SPACE);
|
||||
}
|
||||
|
||||
IR.mark(PANASONIC_AIRCON1_BIT_MARK);
|
||||
IR.space(0);
|
||||
}
|
||||
|
||||
// Send the Panasonic CKP On/Off code
|
||||
//
|
||||
// CKP does not have discrete ON/OFF commands, but this can be emulated by using the timer
|
||||
// The side-effects of using the timer are:
|
||||
// * ONE minute delay before the power state changes
|
||||
// * the 'TIMER' led (orange) is lit
|
||||
// * a timer event is scheduled to cancel the timer after TWO minutes (the 'TIMER' led turns off
|
||||
void PanasonicCKPHeatpumpIR::sendPanasonicCKPOnOffTimerCancel(IRSender& IR, boolean powerState, boolean cancelTimer)
|
||||
{
|
||||
static const prog_uint8_t ON_msg[] PROGMEM = { 0x7F, 0x38, 0xBF, 0x38, 0x10, 0x3D, 0x80, 0x3D, 0x09, 0x34, 0x80, 0x34 }; // ON at 00:10, time now 00:09, no OFF timing
|
||||
static const prog_uint8_t OFF_msg[] PROGMEM = { 0x10, 0x38, 0x80, 0x38, 0x7F, 0x3D, 0xBF, 0x3D, 0x09, 0x34, 0x80, 0x34 }; // OFF at 00:10, time now 00:09, no ON timing
|
||||
static const prog_uint8_t CANCEL_msg[] PROGMEM = { 0x7F, 0x38, 0xBF, 0x38, 0x7F, 0x3D, 0xBF, 0x3D, 0x17, 0x34, 0x80, 0x34 }; // Timer CANCEL
|
||||
|
||||
// Save some SRAM by only having one copy of the template on the SRAM
|
||||
byte sendBuffer[sizeof(ON_msg)];
|
||||
|
||||
if ( cancelTimer == true ) {
|
||||
memcpy_P(sendBuffer, CANCEL_msg, sizeof(ON_msg));
|
||||
} else if ( powerState == true ) {
|
||||
memcpy_P(sendBuffer, ON_msg, sizeof(ON_msg));
|
||||
} else {
|
||||
memcpy_P(sendBuffer, OFF_msg, sizeof(ON_msg));
|
||||
}
|
||||
|
||||
// 40 kHz PWM frequency
|
||||
IR.setFrequency(40);
|
||||
|
||||
for (int i=0; i<6; i++) {
|
||||
IR.mark(PANASONIC_AIRCON1_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_HDR_SPACE);
|
||||
|
||||
IR.sendIRByte(sendBuffer[i*2 + 0], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[i*2 + 0], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[i*2 + 1], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
IR.sendIRByte(sendBuffer[i*2 + 1], PANASONIC_AIRCON1_BIT_MARK, PANASONIC_AIRCON1_ZERO_SPACE, PANASONIC_AIRCON1_ONE_SPACE);
|
||||
|
||||
IR.mark(PANASONIC_AIRCON1_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_HDR_SPACE);
|
||||
|
||||
if ( i < 5 ) {
|
||||
IR.mark(PANASONIC_AIRCON1_BIT_MARK);
|
||||
IR.space(PANASONIC_AIRCON1_MSG_SPACE);
|
||||
}
|
||||
}
|
||||
|
||||
IR.mark(PANASONIC_AIRCON1_BIT_MARK);
|
||||
IR.space(0);
|
||||
}
|
||||
|
||||
// Send the Panasonic CKP timer cancel
|
||||
void PanasonicCKPHeatpumpIR::sendPanasonicCKPCancelTimer(IRSender& IR)
|
||||
{
|
||||
Serial.println(F("Sending Panasonic CKP timer cancel"));
|
||||
|
||||
sendPanasonicCKPOnOffTimerCancel(IR, false, true);
|
||||
}
|
||||
|
|
@ -0,0 +1,57 @@
|
|||
/*
|
||||
Panasonic CKP heatpump control (remote control P/N A75C2295)
|
||||
*/
|
||||
#ifndef PanasonicCKPHeatpumpIR_h
|
||||
#define PanasonicCKPHeatpumpIR_h
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
#include "HeatpumpIR.h"
|
||||
|
||||
|
||||
// Panasonic CKP timing constants (remote control P/N A75C2295)
|
||||
#define PANASONIC_AIRCON1_HDR_MARK 3400
|
||||
#define PANASONIC_AIRCON1_HDR_SPACE 3500
|
||||
#define PANASONIC_AIRCON1_BIT_MARK 800
|
||||
#define PANASONIC_AIRCON1_ONE_SPACE 2700
|
||||
#define PANASONIC_AIRCON1_ZERO_SPACE 1000
|
||||
#define PANASONIC_AIRCON1_MSG_SPACE 14000
|
||||
|
||||
// Panasonic CKP codes
|
||||
#define PANASONIC_AIRCON1_MODE_AUTO 0x06 // Operating mode
|
||||
#define PANASONIC_AIRCON1_MODE_HEAT 0x04
|
||||
#define PANASONIC_AIRCON1_MODE_COOL 0x02
|
||||
#define PANASONIC_AIRCON1_MODE_DRY 0x03
|
||||
#define PANASONIC_AIRCON1_MODE_FAN 0x01
|
||||
#define PANASONIC_AIRCON1_MODE_ONOFF 0x00 // Toggle ON/OFF
|
||||
#define PANASONIC_AIRCON1_MODE_KEEP 0x08 // Do not toggle ON/OFF
|
||||
#define PANASONIC_AIRCON1_FAN_AUTO 0xF0 // Fan speed
|
||||
#define PANASONIC_AIRCON1_FAN1 0x20
|
||||
#define PANASONIC_AIRCON1_FAN2 0x30
|
||||
#define PANASONIC_AIRCON1_FAN3 0x40
|
||||
#define PANASONIC_AIRCON1_FAN4 0x50
|
||||
#define PANASONIC_AIRCON1_FAN5 0x60
|
||||
#define PANASONIC_AIRCON1_VS_SWING 0xF0 // Vertical swing
|
||||
#define PANASONIC_AIRCON1_VS_UP 0x90
|
||||
#define PANASONIC_AIRCON1_VS_MUP 0xA0
|
||||
#define PANASONIC_AIRCON1_VS_MIDDLE 0xB0
|
||||
#define PANASONIC_AIRCON1_VS_MDOWN 0xC0
|
||||
#define PANASONIC_AIRCON1_VS_DOWN 0xD0
|
||||
#define PANASONIC_AIRCON1_HS_SWING 0x08 // Horizontal swing
|
||||
#define PANASONIC_AIRCON1_HS_MANUAL 0x00
|
||||
|
||||
|
||||
class PanasonicCKPHeatpumpIR : public HeatpumpIR
|
||||
{
|
||||
public:
|
||||
PanasonicCKPHeatpumpIR();
|
||||
void send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd);
|
||||
void sendPanasonicCKPCancelTimer(IRSender& IR);
|
||||
|
||||
private:
|
||||
void sendPanasonicCKP(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature, byte swingV, byte swingH);
|
||||
void sendPanasonicCKPraw(IRSender& IR, byte sendBuffer[]);
|
||||
void sendPanasonicCKPOnOffTimerCancel(IRSender& IR, boolean powerState, boolean cancelTimer);
|
||||
};
|
||||
|
||||
#endif
|
|
@ -0,0 +1,218 @@
|
|||
#include <Arduino.h>
|
||||
#include "PanasonicHeatpumpIR.h"
|
||||
|
||||
// This is a protected method, i.e. generic Panasonic instances cannot be created
|
||||
PanasonicHeatpumpIR::PanasonicHeatpumpIR()
|
||||
{
|
||||
}
|
||||
|
||||
// The different models just set the model accordingly
|
||||
PanasonicDKEHeatpumpIR::PanasonicDKEHeatpumpIR()
|
||||
{
|
||||
_panasonicModel = PANASONIC_DKE;
|
||||
}
|
||||
|
||||
PanasonicNKEHeatpumpIR::PanasonicNKEHeatpumpIR()
|
||||
{
|
||||
_panasonicModel = PANASONIC_NKE;
|
||||
}
|
||||
|
||||
PanasonicJKEHeatpumpIR::PanasonicJKEHeatpumpIR()
|
||||
{
|
||||
_panasonicModel = PANASONIC_JKE;
|
||||
}
|
||||
|
||||
// Panasonic DKE/NKE/JKE numeric values to command bytes
|
||||
void PanasonicHeatpumpIR::send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd)
|
||||
{
|
||||
// Sensible defaults for the heat pump mode
|
||||
|
||||
byte operatingMode = PANASONIC_AIRCON2_TIMER_CNL;
|
||||
byte fanSpeed = PANASONIC_AIRCON2_FAN_AUTO;
|
||||
byte temperature = 23;
|
||||
byte swingV = PANASONIC_AIRCON2_VS_UP;
|
||||
byte swingH = PANASONIC_AIRCON2_HS_AUTO;
|
||||
|
||||
switch (powerModeCmd)
|
||||
{
|
||||
case POWER_ON:
|
||||
operatingMode |= PANASONIC_AIRCON2_MODE_ON;
|
||||
break;
|
||||
}
|
||||
|
||||
switch (operatingModeCmd)
|
||||
{
|
||||
case MODE_AUTO:
|
||||
operatingMode |= PANASONIC_AIRCON2_MODE_AUTO;
|
||||
break;
|
||||
case MODE_HEAT:
|
||||
operatingMode |= PANASONIC_AIRCON2_MODE_HEAT;
|
||||
break;
|
||||
case MODE_COOL:
|
||||
operatingMode |= PANASONIC_AIRCON2_MODE_COOL;
|
||||
break;
|
||||
case MODE_DRY:
|
||||
operatingMode |= PANASONIC_AIRCON2_MODE_DRY;
|
||||
break;
|
||||
case MODE_FAN:
|
||||
operatingMode |= PANASONIC_AIRCON2_MODE_FAN;
|
||||
temperatureCmd = 27; // Temperature is always 27 in FAN mode
|
||||
break;
|
||||
case MODE_MAINT: // Maintenance mode is just the heat mode at +8 or +10, FAN5
|
||||
operatingMode |= PANASONIC_AIRCON2_MODE_HEAT;
|
||||
temperature = 10; // Default to +10 degrees
|
||||
fanSpeedCmd = FAN_5;
|
||||
break;
|
||||
}
|
||||
|
||||
switch (fanSpeedCmd)
|
||||
{
|
||||
case FAN_AUTO:
|
||||
fanSpeed = PANASONIC_AIRCON2_FAN_AUTO;
|
||||
break;
|
||||
case FAN_1:
|
||||
fanSpeed = PANASONIC_AIRCON2_FAN1;
|
||||
break;
|
||||
case FAN_2:
|
||||
fanSpeed = PANASONIC_AIRCON2_FAN2;
|
||||
break;
|
||||
case FAN_3:
|
||||
fanSpeed = PANASONIC_AIRCON2_FAN3;
|
||||
break;
|
||||
case FAN_4:
|
||||
fanSpeed = PANASONIC_AIRCON2_FAN4;
|
||||
break;
|
||||
case FAN_5:
|
||||
fanSpeed = PANASONIC_AIRCON2_FAN5;
|
||||
break;
|
||||
}
|
||||
|
||||
if ( temperatureCmd > 15 && temperatureCmd < 31)
|
||||
{
|
||||
temperature = temperatureCmd;
|
||||
}
|
||||
|
||||
switch (swingVCmd)
|
||||
{
|
||||
case VDIR_AUTO:
|
||||
case VDIR_SWING:
|
||||
swingV = PANASONIC_AIRCON2_VS_AUTO;
|
||||
break;
|
||||
case VDIR_UP:
|
||||
swingV = PANASONIC_AIRCON2_VS_UP;
|
||||
break;
|
||||
case VDIR_MUP:
|
||||
swingV = PANASONIC_AIRCON2_VS_MUP;
|
||||
break;
|
||||
case VDIR_MIDDLE:
|
||||
swingV = PANASONIC_AIRCON2_VS_MIDDLE;
|
||||
break;
|
||||
case VDIR_MDOWN:
|
||||
swingV = PANASONIC_AIRCON2_VS_MDOWN;
|
||||
break;
|
||||
case VDIR_DOWN:
|
||||
swingV = PANASONIC_AIRCON2_VS_DOWN;
|
||||
break;
|
||||
}
|
||||
|
||||
switch (swingHCmd)
|
||||
{
|
||||
case HDIR_AUTO:
|
||||
case HDIR_SWING:
|
||||
swingH = PANASONIC_AIRCON2_HS_AUTO;
|
||||
break;
|
||||
case HDIR_MIDDLE:
|
||||
swingH = PANASONIC_AIRCON2_HS_MIDDLE;
|
||||
break;
|
||||
case HDIR_LEFT:
|
||||
swingH = PANASONIC_AIRCON2_HS_LEFT;
|
||||
break;
|
||||
case HDIR_MLEFT:
|
||||
swingH = PANASONIC_AIRCON2_HS_MLEFT;
|
||||
break;
|
||||
case HDIR_RIGHT:
|
||||
swingH = PANASONIC_AIRCON2_HS_RIGHT;
|
||||
break;
|
||||
case HDIR_MRIGHT:
|
||||
swingH = PANASONIC_AIRCON2_HS_MRIGHT;
|
||||
break;
|
||||
}
|
||||
|
||||
// NKE has +8 / + 10 maintenance heating, which also means MAX fanspeed
|
||||
if ( _panasonicModel == PANASONIC_NKE )
|
||||
{
|
||||
if ( temperatureCmd == 8 || temperatureCmd == 10 )
|
||||
{
|
||||
temperature = temperatureCmd;
|
||||
fanSpeed = PANASONIC_AIRCON2_FAN5;
|
||||
}
|
||||
}
|
||||
|
||||
sendPanasonic(IR, operatingMode, fanSpeed, temperature, swingV, swingH);
|
||||
}
|
||||
|
||||
// Send the Panasonic DKE/JKE/NKE code
|
||||
void PanasonicHeatpumpIR::sendPanasonic(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature, byte swingV, byte swingH)
|
||||
{
|
||||
// Only bytes 13, 14, 16, 17 and 26 are modified, DKE and JKE seem to share the same template?
|
||||
static const prog_uint8_t panasonicProgmemTemplate[][27] PROGMEM = {
|
||||
// DKE, model 0
|
||||
{ 0x02, 0x20, 0xE0, 0x04, 0x00, 0x00, 0x00, 0x06, 0x02, 0x20, 0xE0, 0x04, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, 0x00, 0x0E, 0xE0, 0x00, 0x00, 0x01, 0x00, 0x06, 0x00 },
|
||||
// JKE, model 1
|
||||
{ 0x02, 0x20, 0xE0, 0x04, 0x00, 0x00, 0x00, 0x06, 0x02, 0x20, 0xE0, 0x04, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, 0x00, 0x0E, 0xE0, 0x00, 0x00, 0x81, 0x00, 0x00, 0x00 },
|
||||
// NKE, model 2
|
||||
{ 0x02, 0x20, 0xE0, 0x04, 0x00, 0x00, 0x00, 0x06, 0x02, 0x20, 0xE0, 0x04, 0x00, 0x00, 0x00, 0x80, 0x00, 0x06, 0x00, 0x0E, 0xE0, 0x00, 0x00, 0x81, 0x00, 0x00, 0x00 }
|
||||
// 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
|
||||
};
|
||||
|
||||
// Save some SRAM by only having one copy of the template on the SRAM
|
||||
byte panasonicTemplate[27];
|
||||
memcpy_P(panasonicTemplate, panasonicProgmemTemplate[_panasonicModel], sizeof(panasonicTemplate));
|
||||
|
||||
panasonicTemplate[13] = operatingMode;
|
||||
panasonicTemplate[14] = temperature << 1;
|
||||
panasonicTemplate[16] = fanSpeed | swingV;
|
||||
|
||||
// Only the DKE model has a setting for the horizontal air flow
|
||||
if ( _panasonicModel == PANASONIC_DKE) {
|
||||
panasonicTemplate[17] = swingH;
|
||||
}
|
||||
|
||||
// Checksum calculation
|
||||
|
||||
byte checksum = 0xF4;
|
||||
|
||||
for (int i=0; i<26; i++) {
|
||||
checksum += panasonicTemplate[i];
|
||||
}
|
||||
|
||||
panasonicTemplate[26] = checksum;
|
||||
|
||||
// 40 kHz PWM frequency
|
||||
IR.setFrequency(40);
|
||||
|
||||
// Header
|
||||
IR.mark(PANASONIC_AIRCON2_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON2_HDR_SPACE);
|
||||
|
||||
// First 8 bytes
|
||||
for (int i=0; i<8; i++) {
|
||||
IR.sendIRByte(panasonicTemplate[i], PANASONIC_AIRCON2_BIT_MARK, PANASONIC_AIRCON2_ZERO_SPACE, PANASONIC_AIRCON2_ONE_SPACE);
|
||||
}
|
||||
|
||||
// Pause
|
||||
IR.mark(PANASONIC_AIRCON2_BIT_MARK);
|
||||
IR.space(PANASONIC_AIRCON2_MSG_SPACE);
|
||||
|
||||
// Header
|
||||
IR.mark(PANASONIC_AIRCON2_HDR_MARK);
|
||||
IR.space(PANASONIC_AIRCON2_HDR_SPACE);
|
||||
|
||||
// Last 19 bytes
|
||||
for (int i=8; i<27; i++) {
|
||||
IR.sendIRByte(panasonicTemplate[i], PANASONIC_AIRCON2_BIT_MARK, PANASONIC_AIRCON2_ZERO_SPACE, PANASONIC_AIRCON2_ONE_SPACE);
|
||||
}
|
||||
|
||||
IR.mark(PANASONIC_AIRCON2_BIT_MARK);
|
||||
IR.space(0);
|
||||
}
|
|
@ -0,0 +1,86 @@
|
|||
/*
|
||||
Panasonic DKE/JKE/NKE heatpump control (DKE remote control P/N A75C2616 etc)
|
||||
*/
|
||||
#ifndef PanasonicHeatpumpIR_h
|
||||
#define PanasonicHeatpumpIR_h
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "IRSender.h"
|
||||
#include "HeatpumpIR.h"
|
||||
|
||||
|
||||
// Panasonic DKE, JKE & NKE timing constants (DKE remote control P/N A75C2616)
|
||||
#define PANASONIC_AIRCON2_HDR_MARK 3500
|
||||
#define PANASONIC_AIRCON2_HDR_SPACE 1800
|
||||
#define PANASONIC_AIRCON2_BIT_MARK 420
|
||||
#define PANASONIC_AIRCON2_ONE_SPACE 1350
|
||||
#define PANASONIC_AIRCON2_ZERO_SPACE 470
|
||||
#define PANASONIC_AIRCON2_MSG_SPACE 10000
|
||||
|
||||
// Panasonic DKE, JNE & NKE codes
|
||||
#define PANASONIC_AIRCON2_MODE_AUTO 0x00 // Operating mode
|
||||
#define PANASONIC_AIRCON2_MODE_HEAT 0x40
|
||||
#define PANASONIC_AIRCON2_MODE_COOL 0x30
|
||||
#define PANASONIC_AIRCON2_MODE_DRY 0x20
|
||||
#define PANASONIC_AIRCON2_MODE_FAN 0x60
|
||||
#define PANASONIC_AIRCON2_MODE_OFF 0x00 // Power OFF
|
||||
#define PANASONIC_AIRCON2_MODE_ON 0x01
|
||||
#define PANASONIC_AIRCON2_TIMER_CNL 0x08
|
||||
#define PANASONIC_AIRCON2_FAN_AUTO 0xA0 // Fan speed
|
||||
#define PANASONIC_AIRCON2_FAN1 0x30
|
||||
#define PANASONIC_AIRCON2_FAN2 0x40
|
||||
#define PANASONIC_AIRCON2_FAN3 0x50
|
||||
#define PANASONIC_AIRCON2_FAN4 0x60
|
||||
#define PANASONIC_AIRCON2_FAN5 0x70
|
||||
#define PANASONIC_AIRCON2_VS_AUTO 0x0F // Vertical swing
|
||||
#define PANASONIC_AIRCON2_VS_UP 0x01
|
||||
#define PANASONIC_AIRCON2_VS_MUP 0x02
|
||||
#define PANASONIC_AIRCON2_VS_MIDDLE 0x03
|
||||
#define PANASONIC_AIRCON2_VS_MDOWN 0x04
|
||||
#define PANASONIC_AIRCON2_VS_DOWN 0x05
|
||||
#define PANASONIC_AIRCON2_HS_AUTO 0x0D // Horizontal swing
|
||||
#define PANASONIC_AIRCON2_HS_MIDDLE 0x06
|
||||
#define PANASONIC_AIRCON2_HS_LEFT 0x09
|
||||
#define PANASONIC_AIRCON2_HS_MLEFT 0x0A
|
||||
#define PANASONIC_AIRCON2_HS_MRIGHT 0x0B
|
||||
#define PANASONIC_AIRCON2_HS_RIGHT 0x0C
|
||||
|
||||
// Panasonic message templates
|
||||
#define PANASONIC_DKE 0
|
||||
#define PANASONIC_JKE 1
|
||||
#define PANASONIC_NKE 2
|
||||
|
||||
|
||||
class PanasonicHeatpumpIR : public HeatpumpIR
|
||||
{
|
||||
protected:
|
||||
PanasonicHeatpumpIR(); // Cannot create generic Panasonic heatpump instances
|
||||
byte _panasonicModel; // Tells whether this is DKE, NKE or JKE (or other supported model...)
|
||||
|
||||
public:
|
||||
void send(IRSender& IR, byte powerModeCmd, byte operatingModeCmd, byte fanSpeedCmd, byte temperatureCmd, byte swingVCmd, byte swingHCmd);
|
||||
|
||||
private:
|
||||
void sendPanasonic(IRSender& IR, byte operatingMode, byte fanSpeed, byte temperature, byte swingV, byte swingH);
|
||||
};
|
||||
|
||||
class PanasonicDKEHeatpumpIR : public PanasonicHeatpumpIR
|
||||
{
|
||||
public:
|
||||
PanasonicDKEHeatpumpIR();
|
||||
};
|
||||
|
||||
class PanasonicNKEHeatpumpIR : public PanasonicHeatpumpIR
|
||||
{
|
||||
public:
|
||||
PanasonicNKEHeatpumpIR();
|
||||
};
|
||||
|
||||
class PanasonicJKEHeatpumpIR : public PanasonicHeatpumpIR
|
||||
{
|
||||
public:
|
||||
PanasonicJKEHeatpumpIR();
|
||||
};
|
||||
|
||||
|
||||
#endif
|
|
@ -0,0 +1,20 @@
|
|||
arduino-heatpumpir
|
||||
==================
|
||||
|
||||
An Arduino library to control a Panasonic, Midea, Carrier, Fujitsu or Mitsubishi heat pump/split unit air conditioner.
|
||||
Currently supports at least these models
|
||||
* Panasonic E9/E12-CKP (Panasonic remote control P/N A75C2295)
|
||||
* Panasonic E9/E12-DKE (Panasonic remote control P/N A75C2616)
|
||||
* Panasonic E9/E12-JKE and E9/E12-NKE
|
||||
* Midea MSR1-12HRN1-QC2 + MOA1-12HN1-QC2, sold as Ultimate Pro Plus Basic 13FP in Finland (Midea remote control P/N RG51M1/E)
|
||||
* Carrier 42NQV035G / 38NYV035H2 (Carrier remote control P/N WH-L05SE)
|
||||
* Fujitsu Nocria AWYZ14 (remote control P/N AR-PZ2)
|
||||
* Mitsubishi MSZ FD-25, probably also FD-35 (remote control P/N KM09D 0052376)
|
||||
|
||||
|
||||
Instructions
|
||||
------------
|
||||
* Download the library, and place it under your personal Arduino 'libraries' directory, under directory 'HeatpumpIR'
|
||||
* See the example sketches
|
||||
|
||||
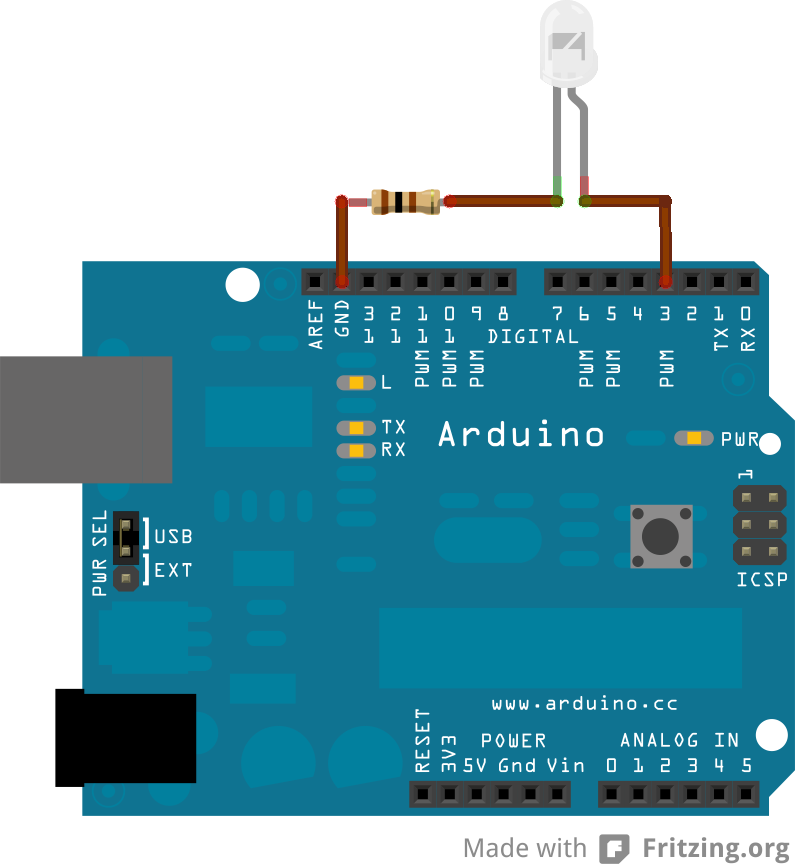
|
Binary file not shown.
After Width: | Height: | Size: 2.6 MiB |
|
@ -0,0 +1,57 @@
|
|||
#include <Arduino.h>
|
||||
#include <PanasonicCKPHeatpumpIR.h>
|
||||
#include <Timer.h>
|
||||
|
||||
/*
|
||||
This schema demonstrates how to control the Panasonic CKP power state change. The CKP does not have discrete
|
||||
'ON' and 'OFF' commands, but only a state switch. So, if the initial power state is now known, switching the
|
||||
state does not help much.
|
||||
|
||||
Luckily this can be implemented by using the timer:
|
||||
* The 'send' command will send all the settings AND program the timer so that the pump will turn ON in a minute
|
||||
* The 'sendPanasonicCKPCancelTimer' will cancel the timer
|
||||
|
||||
The 'turn OFF' must be implemented the same way.
|
||||
|
||||
Of course you can choose to not turn off the timer, but that means that the heatpump will attempt to switch ON
|
||||
(or OFF) every day at the same time, as the timer will still be active.
|
||||
*/
|
||||
|
||||
|
||||
IRSender irSender(3); // IR led on Duemilanove digital pin 3
|
||||
|
||||
PanasonicCKPHeatpumpIR *heatpumpIR;
|
||||
|
||||
Timer timer;
|
||||
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(9600);
|
||||
delay(500);
|
||||
|
||||
heatpumpIR = new PanasonicCKPHeatpumpIR();
|
||||
|
||||
Serial.println("Turning the Panasonic CKP heatpump ON by using the timer");
|
||||
heatpumpIR->send(irSender, POWER_ON, MODE_HEAT, FAN_2, 24, VDIR_UP, HDIR_AUTO);
|
||||
Serial.println("The heatpump should have beeped, and the TIMER led should be ON");
|
||||
|
||||
timer.after(60000, panasonicIsOn); // Called after 1 minute
|
||||
timer.after(120000, panasonicCancelTimer); // Called after 2 minutes
|
||||
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
timer.update();
|
||||
}
|
||||
|
||||
void panasonicIsOn()
|
||||
{
|
||||
Serial.println("The heatpump should should turn ON by now, the TIMER led is still ON");
|
||||
}
|
||||
|
||||
void panasonicCancelTimer()
|
||||
{
|
||||
heatpumpIR->sendPanasonicCKPCancelTimer(irSender);
|
||||
Serial.println("The TIMER led should now be OFF");
|
||||
}
|
|
@ -0,0 +1,37 @@
|
|||
#include <Arduino.h>
|
||||
|
||||
#include "FujitsuHeatpumpIR.h"
|
||||
#include "PanasonicCKPHeatpumpIR.h"
|
||||
#include "PanasonicHeatpumpIR.h"
|
||||
#include "CarrierHeatpumpIR.h"
|
||||
#include "MideaHeatpumpIR.h"
|
||||
#include "MitsubishiHeatpumpIR.h"
|
||||
|
||||
IRSender irSender(3); // IR led on Duemilanove digital pin 3
|
||||
|
||||
// Array with all supported heatpumps
|
||||
HeatpumpIR *heatpumpIR[] = {new PanasonicCKPHeatpumpIR(), new PanasonicDKEHeatpumpIR(), new PanasonicJKEHeatpumpIR(),
|
||||
new PanasonicNKEHeatpumpIR(), new CarrierHeatpumpIR(), new MideaHeatpumpIR(),
|
||||
new FujitsuHeatpumpIR(), new MitsubishiHeatpumpIR(), NULL};
|
||||
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(9600);
|
||||
delay(500);
|
||||
|
||||
Serial.println("Starting");
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
int i = 0;
|
||||
|
||||
do {
|
||||
// Send the same IR command to all supported heatpumps
|
||||
heatpumpIR[i]->send(irSender, POWER_ON, MODE_HEAT, FAN_2, 24, VDIR_UP, HDIR_AUTO);
|
||||
delay(500);
|
||||
}
|
||||
while (heatpumpIR[++i] != NULL);
|
||||
|
||||
delay(2000);
|
||||
}
|
|
@ -0,0 +1,20 @@
|
|||
HeatpumpIR KEYWORD1
|
||||
PanasonicCKPHeatpumpIR KEYWORD1
|
||||
PanasonicDKEHeatpumpIR KEYWORD1
|
||||
PanasonicJKEHeatpumpIR KEYWORD1
|
||||
PanasonicNKEHeatpumpIR KEYWORD1
|
||||
CarrierHeatpumpIR KEYWORD1
|
||||
MideaHeatpumpIR KEYWORD1
|
||||
FujitsuHeatpumpIR KEYWORD1
|
||||
MitsubishiHeatpumpIR KEYWORD1
|
||||
|
||||
IRSender KEYWORD1
|
||||
|
||||
setFrequency KEYWORD2
|
||||
sendIRByte KEYWORD2
|
||||
bitReverse KEYWORD2
|
||||
space KEYWORD2
|
||||
mark KEYWORD2
|
||||
|
||||
send KEYWORD2
|
||||
sendPanasonicCKPCancelTimer KEYWORD2
|
Loading…
Reference in New Issue